Over the last 7 weeks, I have spent lots of time working on finalizing a design of a frequency reactive light panel, and here I have the final results of this project.adidas yeezy 700 v3 nike air max 1 ultra moire black white ราคา shampoo isdin lambdapil Mexico ciorapi compresivi pana la coapsa scarpe eleganti senza lacci nike air max 1 ultra moire black white ราคา дамски памучен чорапогащник plavky chlapec 128nove játék hajszárító árukeresö vans chima ferguson pro 2 port royale black forty two skateboard shop jayden daniels jersey játék hajszárító árukeresö balenciaga 2017 shoes balenciaga 2017 shoes cheap jordan 4
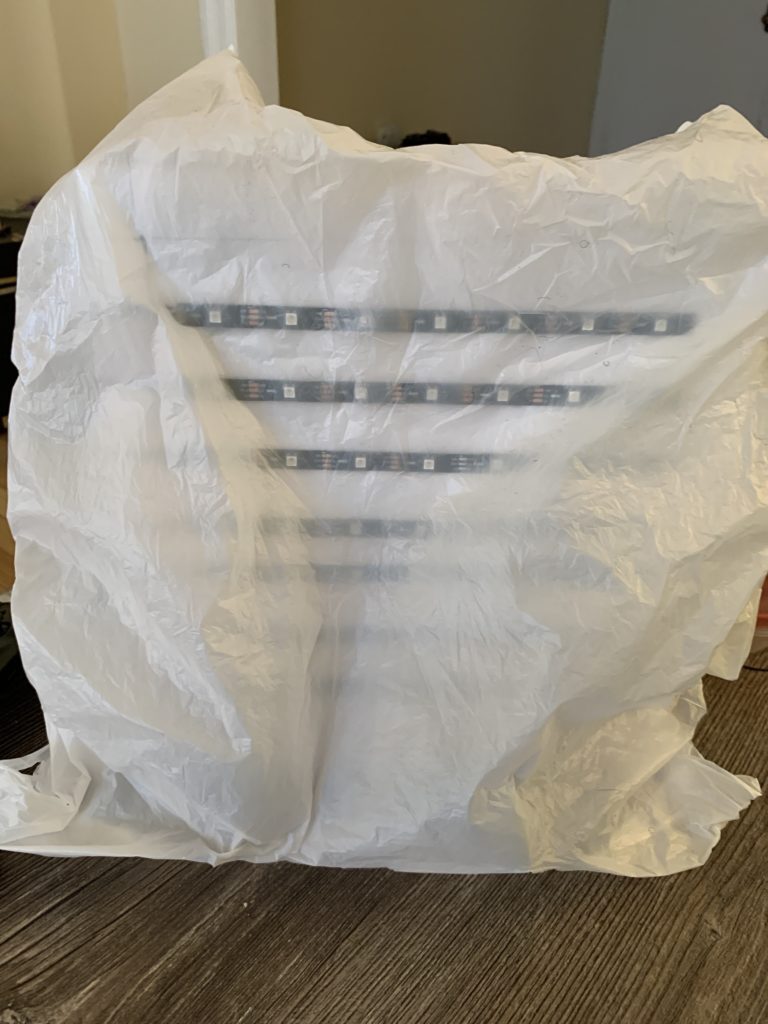
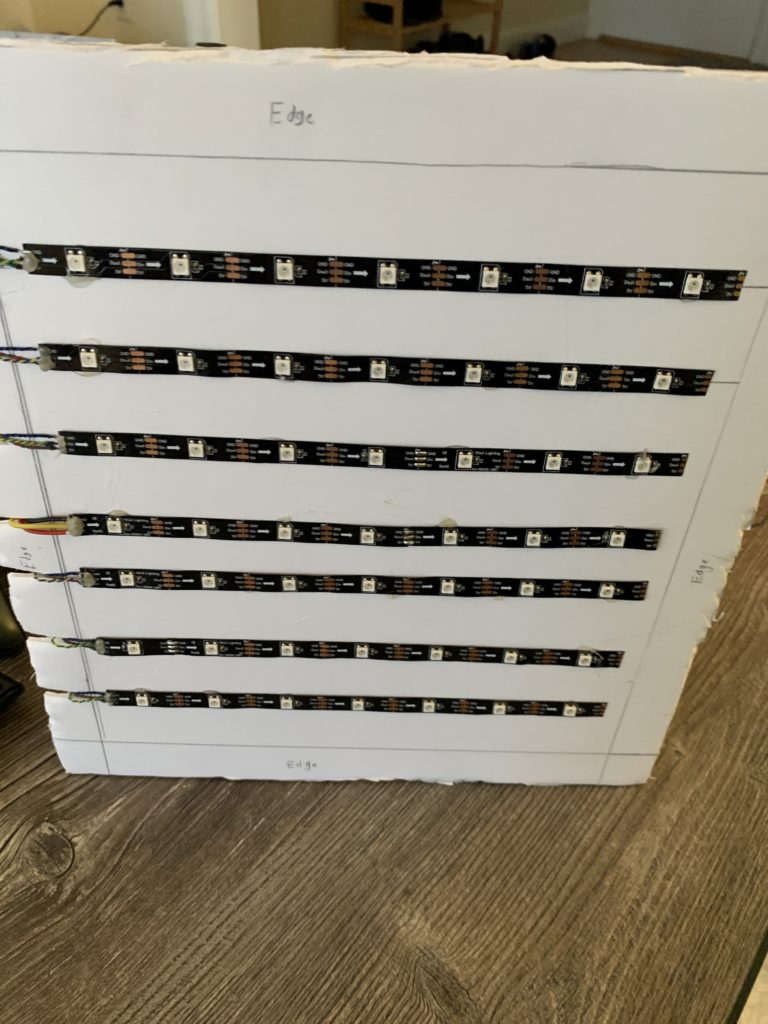
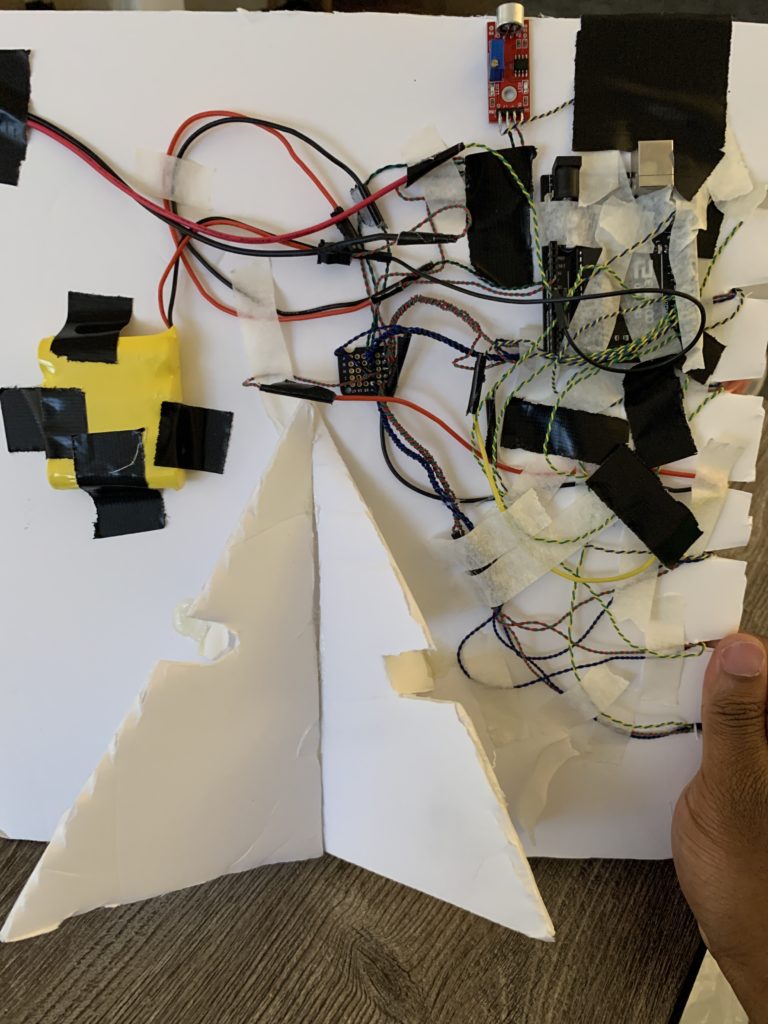
After finalizing the frequency code, I was able to develop vanhunks kayak adidas shoes new design vintage adidas womens sneakers chia seeds benefits for women rochie plaja tricotata cu gauri bej adidas superstar ii femme κρεβατια μονα με αποθηκευτικο χωρο και στρωμα blow up two person kayak une sneaker vans sandals australia workout spandex shorts cazadora estilo motero hombre mug bedrucken amazon amazon goldkette 24 karat herren westerm kalapa fully operational system that reacts based on frequency readings of any sound recieved by the microphone sensor.
Lights and Sensors
Most of the light and sensor programing came easily after finalizing my frequency reading program. One of my trickiest problems was managing my code to allow for frequency detection while also allowing those frequencies to dictate which functions would be run correctly. During my time coding the sensors, I needed to identify several reasons as to why my code would not process a frequency system. After several adjustments, I eventually was able to make the code work successfully, and it would allow for the various lights to succeed.
Following this, I decided to focus on programming my LEDs to use sevaral itterations of code and tested them in several sequences. All code libraries and programs used in this project, and modified variants can be found in the link here. For my final code, I used five functions: an Red, Green, Blue fade in and fade out system, a random color appear and disappear circuit, a meteor trail system that flows light in one direction similar to a meteor trail, and a cyclon style pattern with a random color selected, and a custom burst circuit that utilizes parts of the fade code. Each function was set to run based on a frequency heard between 300hz and 700hz. Below is a demonstration of the LEDs and programs utilized before installation on the final project board
Final Product
After finalizing my code, I was able to get my code into a usable state and attached everything to a piece of posterboard and stood it up with other pieces cut from the same board. Everything was tested with the microphone sensor and succeeded in running the code based on any frequency heard As for a light diffuser, I did not have enough time to get any additional material, so I had to utilize a white plastic bag as the diffuser as it was enough to diffuse light effectively, and was also very useful as a carrying case when not in use. Below is a demonstration of the final product without the cover and with the cover.
After enough work, I was able to deduct that whistling would be the best course of action for showing how the device works, as it is easier to reach certain pitches. However, it is still difficult to get a specific frequency to be noticed by the device with only whistling, and it is best to use a frequency generator to get an exact function.
Final Thoughts and Revisons
After lots of time and effort, the project did succeed, but there is still plenty I wanted to do. For example, I wanted to use this code with other pieces, but these eventually failed as it led to LED issues that would freeze the code and reset the loop earlier than planned. For the project to succeed, I had to only utilize five programs listed as the flash memory of my project was limited due to a mass amount of global variables and several arrays. Additionally, I wanted to make it so that each light strip used could run it’s own individual program. However, this was also something I couldn’t complete due to limitations of the Arduino microcontroller’s flash memory of only 2GB. Due to this, I could not create additional independent arrays without creating more issues for the code, so I had to utilize a linked system to complete this process. Given this, in the future I would prefer to use a microcontroller with a larger amount of flash memory to allow for individual array control. And with that, this brings the end of my final project.
Code
Primary Code
/* Tyson Wiseman - HUA3901 Practicum - Light Art D term
Final Project Room: FLA17
Code: Sound Reactive Light Circuit
Libraries used:
arduinoFFT.h
FastLED.h
meteorTrail
cyclon
Various custom programs:
Burst
Random Rainbow
This project was originally supposed to use multiple arrays for variable control of the LED strips
Unfortunately, due to the dynamic memory constraints, I needed to reduce how many arrays existed. The result is the current model.
For future reference, if I want to control a 7x7 board and be able to program each individual strip unlinked, then I would need more dynamic memory.
*/
//sound code initialization
#include <arduinoFFT.h>
#define SAMPLES 128 //samples-pt FFT
#define SAMPLING_FREQUENCY 2048 //Ts = Based on Nyquist
arduinoFFT FFT = arduinoFFT();
unsigned int samplingPeriod;
unsigned long microSeconds;
double vReal[SAMPLES]; //vector of size SAMPLES to hold real vals
double vImag[SAMPLES]; // vector of size SAMPLES to hold imaginary values
double peak;
//LED strip initalization
#include <FastLED.h>
//define LED Strips
#define LED0 2
#define LED1 3
#define LED2 4
#define LED3 5
#define LED4 6
#define LED5 7
#define LED6 8
#define NUM_LEDS 7
//define RGB Byte for Randomizer
byte r, g, b;
//linked array
CRGB leds[NUM_LEDS];
//define sound sensor
const int micPin = A0;
//state machine prep
int mainState = 0;
void setup() {
//initialize serial monitor
Serial.begin(115200);
//initialize frequency timer
samplingPeriod = round(1000000 * (1.0 / SAMPLING_FREQUENCY)); //period is microseconds
//initialize LED strips
{ //strip 0
FastLED.addLeds<WS2812, LED0, GRB>(leds, NUM_LEDS);
}
{ //strip 1
FastLED.addLeds<WS2812, LED1, GRB>(leds, NUM_LEDS);
}
{ //strip 2
FastLED.addLeds<WS2812, LED2, GRB>(leds, NUM_LEDS);
}
{ //strip 3
FastLED.addLeds<WS2812, LED3, GRB>(leds, NUM_LEDS);
}
{ //strip 4
FastLED.addLeds<WS2812, LED4, GRB>(leds, NUM_LEDS);
}
{ //strip 5
FastLED.addLeds<WS2812, LED5, GRB>(leds, NUM_LEDS);
}
{ //strip 6
FastLED.addLeds<WS2812, LED6, GRB>(leds, NUM_LEDS);
}
//Clear the LED strips
setAll(0, 0, 0);
delay(500);
Serial.println("Begin State Machine");
}
//initalize several programs for the code to run
//first light set
void p1 () {
RGBLoop();
}
//random appear
void p2 () {
for (int cur = 0; cur < NUM_LEDS; cur++) {
// chose random value for the r/g/b
r = random(0, 255);
g = random(0, 255);
b = random(0, 255);
//set the value to the led
leds[cur] = CRGB (r, g, b);
// set the colors set into the phisical LED
FastLED.show();
// delay 50 millis
FastLED.delay(200);
}
for (int fin = 0; fin < NUM_LEDS; fin++) {
// chose random value for the r/g/b
//set the value to the led
leds[fin] = CRGB (0, 0, 0);
// set the colors set into the phisical LED
FastLED.show();
// delay 50 millis
FastLED.delay(200);
}
}
//meteor rain
void p3 () {
meteorRain(random(0, 255), random(0, 255), random(0, 255), 1, 200, false, 60);
}
//cyclon
void p4 () {
CylonBounce(random(0, 255), random(0, 255), random(0, 255), 1, 100, 100, 2);
}
//burst
void p5 () {
// TwinkleRandom(7, 100, false);
burst(1);
}
//main decision code
void lights(double peak = FFT.MajorPeak(vReal, SAMPLES, SAMPLING_FREQUENCY)) {
int pReal = peak - 10;
if (pReal < 400 && pReal > 300) {
Serial.print("begin p1...");
p1();
Serial.println("Complete");
Serial.println();
delay(250);
}
else if (pReal > 400 && pReal < 500) {
Serial.print("begin p2...");
p2();
Serial.println("Complete");
Serial.println();
delay(250);
}
else if (pReal < 600 && pReal > 500) {
Serial.print("begin p3...");
p3();
Serial.println("Complete");
Serial.println();
delay(250);
}
else if (pReal > 600 && pReal < 700) {
Serial.print("begin p4...");
p4();
Serial.println("Complete");
Serial.println();
delay(250);
}
else if (pReal > 700) {
Serial.print("begin p5...");
p5();
Serial.println("Complete");
Serial.println();
delay(250);
}
else {
Serial.println("nothing fits, return to other code");
Serial.println();
delay(250);
return;
}
}
void loop() {
// put your main code here, to run repeatedly:
switch (mainState) {
case 0:
frequency();
Serial.println(" Frequency determined. Move to lights");
Serial.println();
mainState = 1;
delay(10);
break;
case 1:
lights(peak);
Serial.println("return to frequency");
Serial.println();
mainState = 0;
break;
default:
break;
}
}
//Simplified pixel controller
//show the strips
void showStrip() {
#ifndef ADAFRUIT_NEOPIXEL_H
// FastLED
FastLED.show();
#endif
}
//set a pixel for all strips
void setPixel(int Pixel, byte red, byte green, byte blue) {
#ifndef ADAFRUIT_NEOPIXEL_H
// FastLED
//all strips
leds[Pixel].r = red;
leds[Pixel].g = green;
leds[Pixel].b = blue;
#endif
}
//set all pixels
void setAll(byte red, byte green, byte blue) {
for (int i = 0; i < NUM_LEDS; i++ ) {
setPixel(i, red, green, blue);
}
showStrip();
}
Fade Code
void RGBLoop(){
for(int j = 0; j < 3; j++ ) {
// Fade IN
for(int k = 0; k < 256; k++) {
switch(j) {
case 0: setAll(k,0,0); break;
case 1: setAll(0,k,0); break;
case 2: setAll(0,0,k); break;
}
//showStrip();
}
// Fade OUT
for(int k = 255; k >= 0; k--) {
switch(j) {
case 0: setAll(k,0,0); break;
case 1: setAll(0,k,0); break;
case 2: setAll(0,0,k); break;
}
//showStrip();
}
}
}
Meteor Rain Code
void meteorRain(byte red, byte green, byte blue, byte meteorSize, byte meteorTrailDecay, boolean meteorRandomDecay, int SpeedDelay) {
setAll(0, 0, 0);
for (int i = 0; i < NUM_LEDS + NUM_LEDS; i++) {
// fade brightness all LEDs one step
for (int j = 0; j < NUM_LEDS; j++) {
if ( (!meteorRandomDecay) || (random(10) > 5) ) {
fadeToBlack(j, meteorTrailDecay );
}
}
// draw meteor
for (int j = 0; j < meteorSize; j++) {
if ( ( i - j < NUM_LEDS) && (i - j >= 0) ) {
setPixel(i - j, red, green, blue);
}
}
showStrip();
delay(SpeedDelay);
}
}
void fadeToBlack(int ledNo, byte fadeValue) {
// #ifdef ADAFRUIT_NEOPIXEL_H
// // NeoPixel
// uint32_t oldColor;
// uint8_t r, g, b;
// int value;
//
// oldColor = strip.getPixelColor(ledNo);
// r = (oldColor & 0x00ff0000UL) >> 16;
// g = (oldColor & 0x0000ff00UL) >> 8;
// b = (oldColor & 0x000000ffUL);
//
// r=(r<=10)? 0 : (int) r-(r*fadeValue/256);
// g=(g<=10)? 0 : (int) g-(g*fadeValue/256);
// b=(b<=10)? 0 : (int) b-(b*fadeValue/256);
//
// strip.setPixelColor(ledNo, r,g,b);
// #endif
#ifndef ADAFRUIT_NEOPIXEL_H
// FastLED
leds[ledNo].fadeToBlackBy( fadeValue );
#endif
}
Cyclon Code
void CylonBounce(byte red, byte green, byte blue, int EyeSize, int SpeedDelay, int ReturnDelay, int counter) {
for (int i = 0; i < counter; i++) {
for (int i = 0; i < NUM_LEDS - EyeSize - 2; i++) {
setAll(0, 0, 0);
setPixel(i, red / 10, green / 10, blue / 10);
for (int j = 1; j <= EyeSize; j++) {
setPixel(i + j, red, green, blue);
}
setPixel(i + EyeSize + 1, red / 10, green / 10, blue / 10);
showStrip();
delay(SpeedDelay);
}
delay(ReturnDelay);
for (int i = NUM_LEDS - EyeSize - 2; i > (-1); i--) {
setAll(0, 0, 0);
setPixel(i, red / 10, green / 10, blue / 10);
for (int j = 1; j <= EyeSize; j++) {
setPixel(i + j, red, green, blue);
}
setPixel(i + EyeSize + 1, red / 10, green / 10, blue / 10);
showStrip();
delay(SpeedDelay);
}
delay(ReturnDelay);
setAll(0, 0, 0);
}
}
Burst Code
void burst(int count) {
r = random(0, 255);
g = random(0, 255);
b = random(0, 255);
for (int i = 0; i < count; i++) {
//sparks
setPixel(NUM_LEDS * (0.3), random(0, 255), random(0, 255), random(0, 255));
showStrip();
delay(100);
setAll(0, 0, 0);
delay(100);
setPixel(NUM_LEDS * (0.8), random(0, 255), random(0, 255), random(0, 255));
showStrip();
delay(100);
showStrip();
setAll(0, 0, 0);
delay(100);
setPixel(NUM_LEDS * (0.1), random(0, 255), random(0, 255), random(0, 255));
showStrip();
delay(100);
setAll(0, 0, 0);
delay(100);
//BURST OUT
setPixel(3, r, g, b);
showStrip();
delay(300);
//fade out
for (int j = 0; j < 3; j++ ) {
for (int k = 255; k >= 0; k--) {
switch (j) {
case 0: setAll(k, 0, 0); break;
case 1: setAll(0, k, 0); break;
case 2: setAll(0, 0, k); break;
}
}
}
}
}
I liked the interactive component of this piece. I think it could be cool to whistle certain tones to get different reactions. It could also be interesting to visualize the sound of a noisy room.
The WS2812 LEDs are super bright! If you can also implement controlling the brightness based on the volume it will be extra nice. The last mode is a bit harsh on my eyes but the rest of them looks pretty!
The results of this were really cool and fun. I would love to see more of this being demonstrated, especially with something to diffuse the lights.